You would like to show off your cool Rig in a rendered Shot? Or maybe you would just like to quickly render curves for cables, tubes, etc?
This little script helps with that time consuming task of making every single nurbscurve renderable. Simply select the Rigs/Objects the curves are parented to, and let Python do the heavy lifting.
import maya.cmds as cmds
#get selected objects
selection = cmds.ls( selection=True)
#selecting each and every object in the hierachy
for s in selection:
cmds.select(s, hi=True,add=True)
#get all nurbsCurves
sel = cmds.ls( selection=True, type="nurbsCurve" )
#loop through curves
for s in sel:
#settings for Curve Width should be adjusted to the scaling of your scene.
cmds.setAttr(s+".aiRenderCurve", 1)
cmds.setAttr(s+".aiSampleRate", 120)
cmds.setAttr(s+".aiCurveWidth", 0.015)
cmds.setAttr(s+".castsShadows", 0)
#create Arnold Material
material = cmds.shadingNode("aiStandardSurface", name=s+"_mtl", asShader=True)
sg = cmds.sets(name="%sSG" % s+"_mtl", empty=True, renderable=True, noSurfaceShader=True)
cmds.connectAttr("%s.outColor" % material, "%s.surfaceShader" % sg)
#set material attributes
cmds.setAttr(material+".specular", 0)
cmds.setAttr(material+".base", 1)
#get curve color from index
matcolor = cmds.getAttr(s + ".overrideColor")
try:
matcolor = cmds.colorIndex( matcolor, q=True )
except:
matcolor = [1.0, 1.0, 1.0]
#set color to material
cmds.setAttr(material+".baseColor", matcolor[0] , matcolor[1] , matcolor[2] , type="double3")
try:
#connect material to curve
cmds.connectAttr(material+".outColor", s+".aiCurveShader")
except:
pass
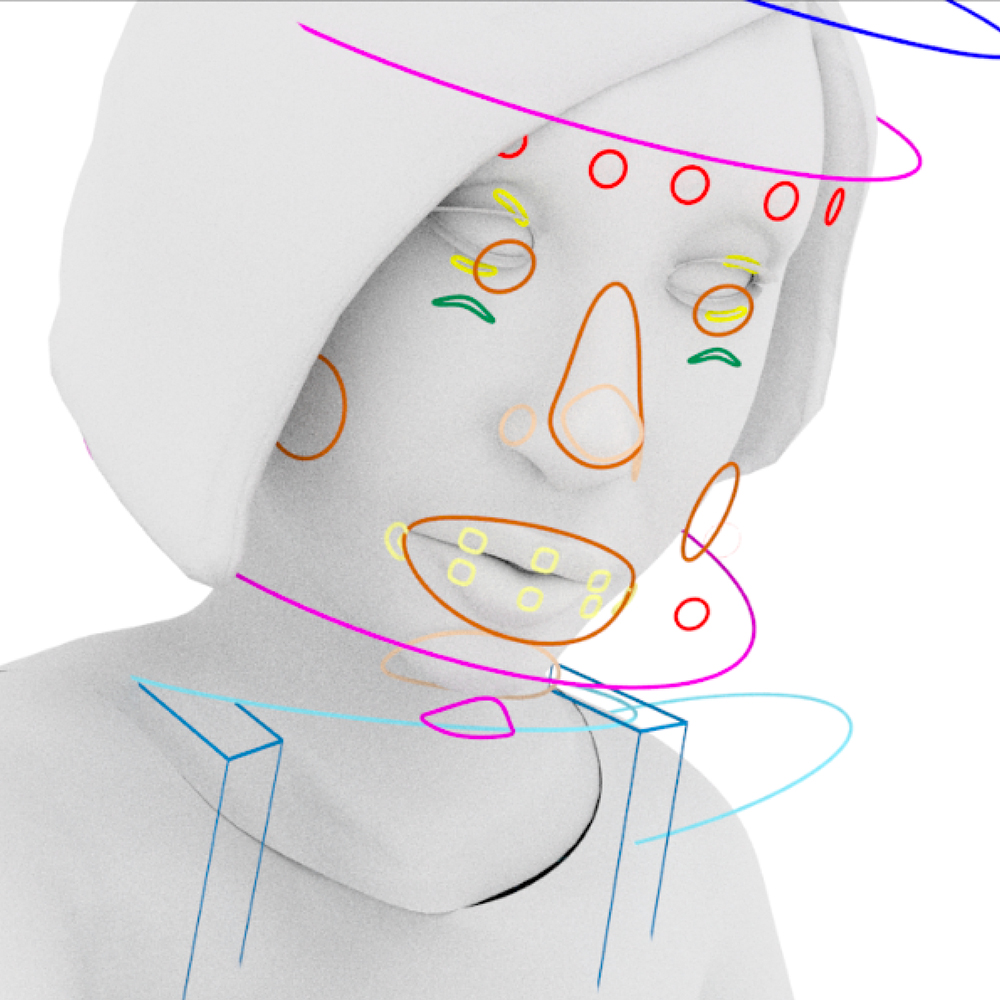